How To Start Website Development
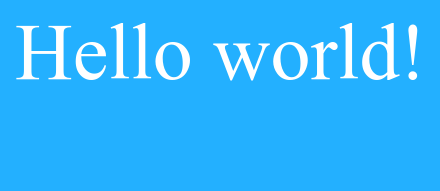
The first time you look at computer code you might stare until you go cross-eyed, but with a little guidance that same mangled text can quickly become a sharp tool for creativity. This introductory guide is something I wish I had when starting out. I hope it can help those with zero experience get started or at least walk away with an understanding of a few concepts found in website development.
Object⌗
an object in programming is a collection of data
const message = {
"sentence": "Hello world!"
}
- const - stands for
constant variable
a variable can typically change its value, but a constant variable will not change.
HTML⌗
HTML stands for Hypertext Markup Language which is in a class of languages called Markup Languages and it tells Web Browsers what to do with your important website information.
<div id="webpage">Hello world</div>
- div - stands for
division
, but not like in math, adiv
tag seperates elements in a page. - This div has an
id
attribute we assign the name of “webpage” for use in our upcoming script. </div>
- every tag in HTML has aclosing tag
represented with the name of the Element with a/
in front, in our case</div>
.
Javascript⌗
Javascript is what’s called a scripting language
This example is actually a subset of Javascript, called a framework, named jQuery. I find it both more efficient and easier to learn Javascript through playing with jQuery, but there are reasons, you’ll discover on your own, for pure Javascript and supersets of Javascript like Typescript
$("#webpage").text(message.sentence);
- The
$()
is ajQuery selector
it’s a shorthand selector for HTML DOM Elements, Classes, and in our case IDs, denoted by the preceeding#
and our<div>
’s given namewebpage
. - The
.
following the$()
is a chain operator, which promises to execute each following function attached, in our case it’stext()
with our Object called. - The Object is in a format called Javascript Object Notation typically shortened to JSON which also uses a
.
operator to denote the hierarchical structure we defined before - Our
message
variable contains thesentence
key which returns theHello world!
value to the<div>
with the ID (#
) ofwebpage
astext()
. This is the essence of data structures in a format called Key-Value Store which will be your bread and butter for all things data going forward.
<filename>.html from a web browser
Putting It All Together!
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Key-Value Store Example</title>
<script src="https://code.jquery.com/jquery-3.7.1.min.js" integrity="sha256-/JqT3SQfawRcv/BIHPThkBvs0OEvtFFmqPF/lYI/Cxo=" crossorigin="anonymous"></script>
</head>
<body style="background:pink">
<!-- HTML -->
<div id="webpage">Hello world</div>
<script>
// Object
const message = {
"sentence": "Hello world!"
};
// Javascript
$("#webpage").text(message.sentence);
</script>
</body>
</html>
- WOW THAT’S A LOT MORE STUFF!
- Now that you understand the basics and the few things that really mattered in this web page, there’s nothing left but to see a real world example such as this.
- There’s a lot here, but one thing to play with is the
style
attribute on the<body>
tag, start by changing that value and have fun learning! - you can copy/paste this code into a file called
hello.html
and open it in your web browser to view. To edit again right click and selectopen with
and choose a text editor. I recommend downloading Microsoft VS Code if you don’t have anything but notepad. - I also recommend typing all of this block out by hand so you can get a feel for it, but also understand that there is tooling out there such as Emmet that are meant to generate stuff for you later on in your journey~
- From here check out the LAMP Stack
- and be sure you have visited W3C Schools
- Happy Hacking!